Developers working with macOS applications face various error messages.
One error that can lead to confusion is associated with the domain NSCocoaErrorDomain is errordomain=nscocoaerrordomain&errormessage=kunde inte hitta den angivna genvägen.&errorcode=4.
This error is identified by its message, “kunde inte hitta den angivna genvägen.&errorcode=4,” which means “Unable to find the specified shortcut.”
It can be particularly frustrating for those who do not speak French fluently.
This article aims to clarify this error, examine its potential causes, and suggest effective solutions for developers facing errordomain=nscocoaerrordomain&errormessage=kunde inte hitta den angivna genvägen.&errorcode=4 error.
Before examining errordomain=nscocoaerrordomain&errormessage=kunde inte hitta den angivna genvägen.&errorcode=4 in detail, it’s important to grasp the meaning of NSCocoaErrorDomain.
In macOS and iOS development, errors are organized into domains that indicate where the error originated.
NSCocoaErrorDomain is a specific domain used by Apple’s Cocoa and Cocoa Touch frameworks. These frameworks are essential for developing applications on macOS and iOS.
Errors that fall under this domain often relate to:
- File management
- Data storage
- Core functionalities provided by these frameworks
What Is errordomain=nscocoaerrordomain&errormessage=kunde inte hitta den angivna genvägen.&errorcode=4?
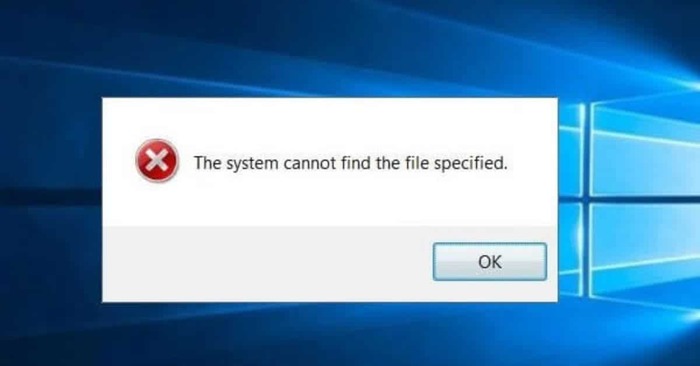
The error message “kunde inte hitta den angivna genvägen.&errorcode=4” translates to “Unable to locate the specified shortcut.”
errordomain=nscocoaerrordomain&errormessage=kunde inte hitta den angivna genvägen.&errorcode=4 typically signals a file not found issue.
This problem can arise in several situations, such as an incorrect file path, a file that has been moved or deleted, or a typo in the file name or path.
How To Fix errordomain=nscocoaerrordomain&errormessage=kunde inte hitta den angivna genvägen.&errorcode=4?
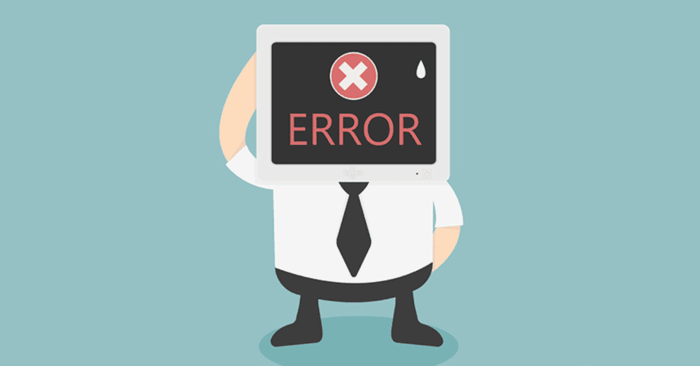
After pinpointing the error’s source, developers can implement the following solutions:
- Utilize Dynamic Path Methods
Refrain from hardcoding file paths within the application. Instead, leverage the framework’s dynamic path resolution techniques, such as NSFileManager in Cocoa. This guarantees that paths are accurately determined based on the current environment and user settings.
- Manage Missing Files Effectively
Integrate error handling to address situations where a file cannot be found. Deliver clear error messages to users and provide alternatives or recovery solutions. For instance, if a configuration file is absent, ask the user to select a new file or revert to default settings.
- Verify User Input
When obtaining file paths from user input, validate the inputs to confirm they are accurate and point to existing files. Give feedback if the entered path needs to be corrected and assist users in making necessary corrections.
- Request Access Permissions
Ensure that the application seeks and secures the required permissions to access files. For applications on macOS, utilize the correct APIs to request file access permissions from users.
Sample Code
To demonstrate how to manage file paths dynamically and incorporate error handling, here’s an example in Swift:
import Foundation
func readFile(from path: String) {
let fileManager = FileManager.default
if fileManager.fileExists(atPath: path) {
do {
let contents = try String(contentsOfFile: path, encoding: .utf8)
print(“File contents: (contents)”)
} catch {
print(“Error while reading file: (error.localizedDescription)”)
}
} else {
print(“Error: The file could not be found at the path (path)”)
}
}
let userFilePath = “/path/to/user/file.txt”
readFile(from: userFilePath)
What Causes errordomain=nscocoaerrordomain&errormessage=kunde inte hitta den angivna genvägen.&errorcode=4 Error?
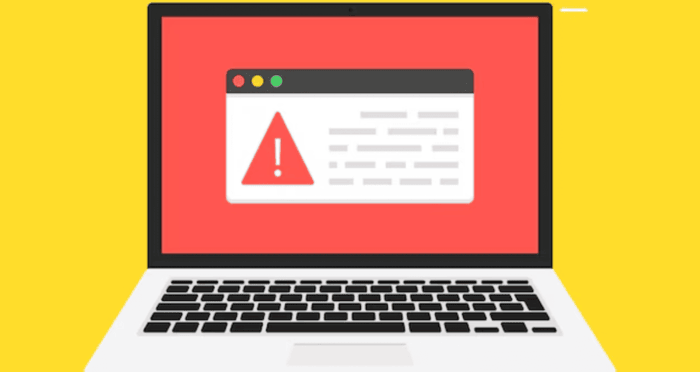
- Invalid File Path
A primary reason for this error is an invalid file path. If the application tries to access a file using a path that does not exist, it will trigger errordomain=nscocoaerrordomain&errormessage=kunde inte hitta den angivna genvägen.&errorcode=4. This often happens when paths are hardcoded without dynamically updating, causing issues when the file structure changes.
- File Relocation or Deletion
This error can also occur if an expected file has been moved or deleted. Changes during updates, user actions, or other applications modifying the file structure can lead to this scenario.
- Spelling Mistakes
Typos in the file name or path can cause this error as well. Even minor errors in the file extension or directory name can prevent the application from finding the required file.
- Access Rights Problems
Sometimes, the error may be linked to inadequate permissions for accessing the specified file. If the application lacks the necessary read or write permissions, it will be unable to find or manipulate the file, resulting in an NSCocoaErrorDomain error.
Identifying the Problem
To effectively fix errordomain=nscocoaerrordomain&errormessage=kunde inte hitta den angivna genvägen.&errorcode=4, developers should adopt a structured method:
- Confirm the File Path
Check that the file path in the code is accurate. Look for errors or hardcoded paths that may not be valid in different settings.
- Validate File Presence
Ensure the file is located at the specified path. Utilize file management tools or terminal commands to verify the file’s availability and accessibility.
- Examine Access Rights
Review the permissions for the file and the directories that lead to it. Ensure the application has the required read and write permissions to access the file.
- Utilize Logging and Debugging
Incorporate logging to gather detailed information regarding attempts to access the file. Employ debugging tools to search the code and pinpoint where the error occurs.
Conclusion
Errordomain=nscocoaerrordomain&errormessage=kunde inte hitta den angivna genvägen.&errorcode=4 presents a significant hurdle for developers working on macOS.
Nevertheless, developers can successfully troubleshoot and fix this issue by grasping its root causes and following best file management and error handling practices.
To create resilient applications, consider the following strategies:
- Dynamically Resolve File Paths
Always ensure that file paths are set up to adapt based on the environment.
- Gracefully Handle Missing Files
Implement user-friendly error messages and recovery options when a file cannot be found.
- Validate Input from Users
Check that all user-provided paths point to valid files.
- Manage Permissions Properly
Make sure your application requests the right permissions for file access.
By employing these techniques, developers can enhance their applications’ reliability regarding file access, ultimately improving the overall user experience.
The post How To Fix errordomain=nscocoaerrordomain&errormessage=kunde inte hitta den angivna genvägen.&errorcode=4 Error? appeared first on About Chromebooks.