Many Android applications require displaying online content within the app itself.
Developers often use WebView or redirect users to an external browser. However, these methods can slow down performance and consume more resources.
A more efficient alternative is to use Custom Chrome Tabs, which allow web pages to open within the application while maintaining smooth performance.
This article explains how Java can integrate Custom Chrome Tabs in an Android application.
Follow the steps carefully to ensure a seamless browsing experience within your app.
Why Use Custom Chrome Tabs?
Using Custom Chrome Tabs offers several benefits:
- Faster page load times than WebView.
- Lighter on system resources since Chrome handles rendering.
- Enhanced security is achieved when browsing within Chrome’s sandboxed environment.
- Familiar UI that retains Chrome’s built-in features, such as autofill and saved passwords.
- Customizable appearance with toolbar color and animations.
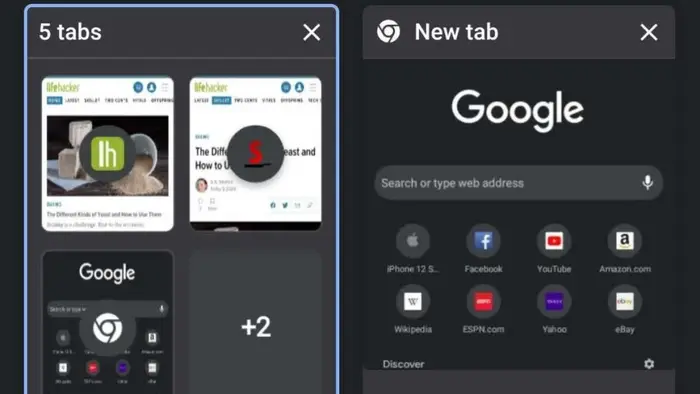
Now, let’s dig into the step-by-step process of integrating Custom Chrome Tabs in an Android application.
Step 1: Create a New Android Project
Start by launching Android Studio and creating a fresh project. Choose Java as the programming language while setting up the environment.
Step 2: Add Browser Dependency
Modify the build.gradle (Module: app) file by adding the required dependency under the dependencies section:
implementation ‘androidx.browser:browser:1.2.0’
Once you insert the dependency, click on Sync Now to apply the changes.
Step 3: Designing the UI Layout
Add a button to the layout file to enable users to open a Custom Chrome Tab. Modify activity_main.xml as follows:
<?xml version=”1.0″ encoding=”utf-8″?>
<RelativeLayout
xmlns:android=”http://schemas.android.com/apk/res/android”
xmlns:tools=”http://schemas.android.com/tools”
android:layout_width=”match_parent”
android:layout_height=”match_parent”
tools:context=”.MainActivity”>
<Button
android:id=”@+id/customTabButton”
android:layout_width=”wrap_content”
android:layout_height=”wrap_content”
android:layout_centerInParent=”true”
android:text=”Launch Custom Tab” />
</RelativeLayout>
Step 4: Implementing Custom Chrome Tabs in Java
Modify the MainActivity.java file to handle button clicks and launch a Custom Chrome Tab when the button is pressed.
import android.app.Activity;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import androidx.appcompat.app.AppCompatActivity;
import androidx.browser.customtabs.CustomTabsIntent;
import androidx.core.content.ContextCompat;
public class MainActivity extends AppCompatActivity {
Button customTabButton;
String webAddress = “https://www.geeksforgeeks.org/”;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
customTabButton = findViewById(R.id.customTabButton);
customTabButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
CustomTabsIntent.Builder tabBuilder = new CustomTabsIntent.Builder();
tabBuilder.setToolbarColor(ContextCompat.getColor(MainActivity.this, R.color.purple_200));
openChromeTab(MainActivity.this, tabBuilder.build(), Uri.parse(webAddress));
}
});
}
public static void openChromeTab(Activity activity, CustomTabsIntent customTabsIntent, Uri uri) {
String chromePackage = “com.android.chrome”;
if (chromePackage != null) {
customTabsIntent.intent.setPackage(chromePackage);
customTabsIntent.launchUrl(activity, uri);
} else {
activity.startActivity(new Intent(Intent.ACTION_VIEW, uri));
}
}
}
Key Features of Custom Chrome Tabs
The implementation of Custom Chrome Tabs utilizes several key elements, which are explained below:
Feature | Description |
CustomTabsIntent.Builder | Constructs an intent to open a Chrome Tab within the app. |
setToolbarColor() | Allows customization of the toolbar color to match the app’s theme. |
launchUrl() | Opens the target URL inside the Chrome Tab. |
setPackage() | Ensures that the tab is launched using the Chrome browser. |
startActivity() | Opens the URL in an external browser if Chrome is unavailable. |
How Custom Chrome Tabs Improve User Experience?
Using Custom Chrome Tabs improves the browsing experience within an Android app by providing a smoother transition between in-app and web-based content.
Unlike WebView, which requires additional configurations and permissions, Custom Chrome Tabs work seamlessly without requiring extra effort from developers.
Here’s why they are a better choice:
- Consistent User Interface: Custom Chrome Tabs retain Chrome’s familiar interface, making search intuitive.
- Improved Performance: Since Chrome handles web page rendering, apps using Custom Chrome Tabs are more responsive and efficient.
- Security Benefits: Pages load securely within Chrome’s protected environment, reducing security risks.
- Customization Options: Developers can personalize the Chrome Tab to match the app’s branding by modifying toolbar colors and animations.
Final Thoughts
Integrating Custom Chrome Tabs is an effective way to display web pages inside an Android application.
This method ensures better performance, improved user experience, and security while keeping resource usage minimal.
Following the steps outlined in this guide, you can successfully implement Custom Chrome Tabs and enhance your app’s browsing capabilities.
By leveraging the capabilities of Custom Chrome Tabs, developers can provide a seamless transition between in-app content and external web pages.
The flexibility to customize its appearance while maintaining Chrome’s built-in security and performance features makes it an ideal choice for modern Android applications.
With this integration, your app will offer a more fluid browsing experience, allowing users to search through the external content without leaving the application.
The implementation process is straightforward, and the benefits far outweigh the minimal effort required to set it up.